Web Component - Get Paid by Apple Pay
Implement Apple Pay in a Headless way in your web page to allow your customers to complete a payment flow with ease
π Introduction
In this guide you'll learn how to add the Apple Pay button to your website!
This guide will walk you through the process of adding the Apple Pay button to your website using NoFrixion WebComponents. With this seamless integration, you'll be able to offer your customers a convenient and secure way to make payments using Apple Pay on your website.
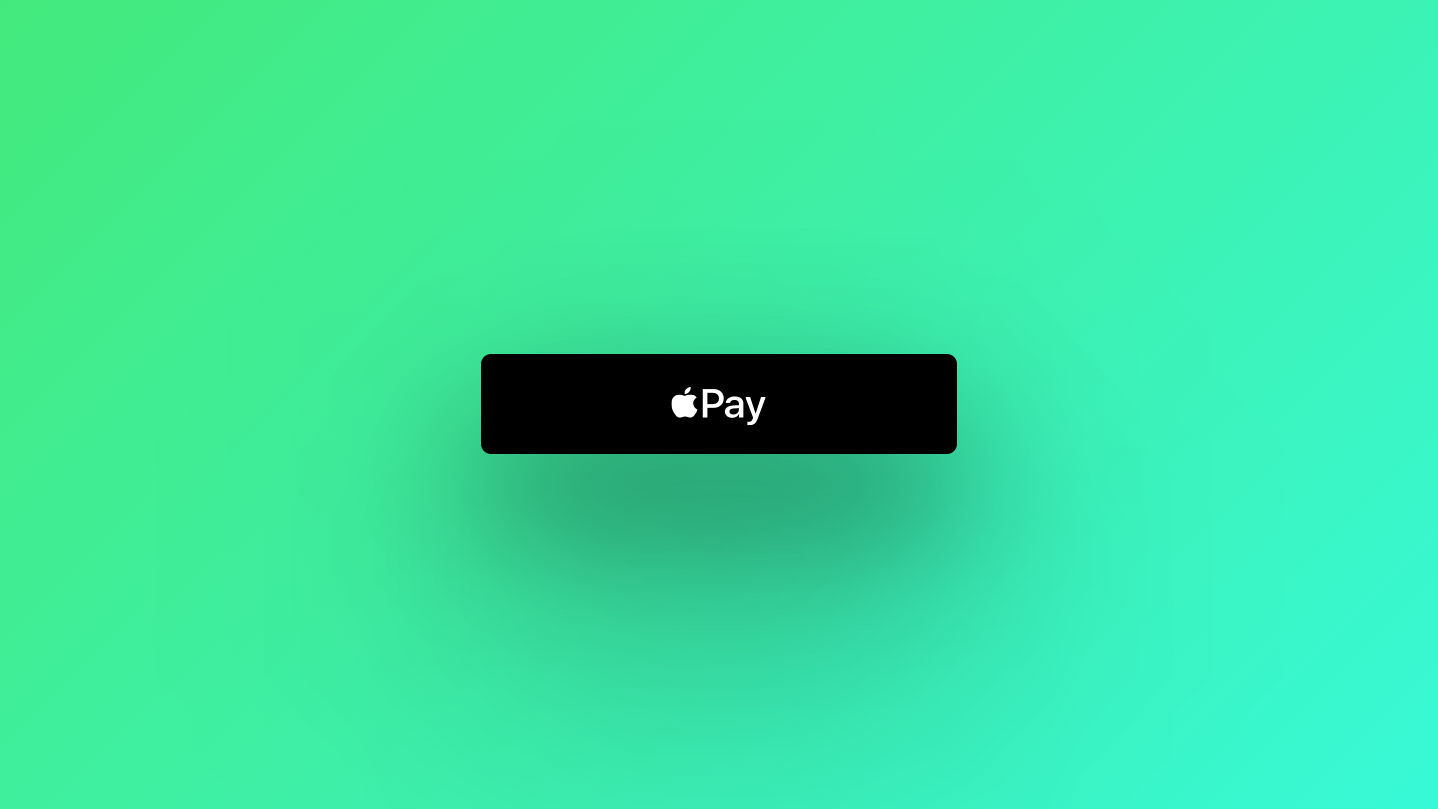
This is how the Apple Pay button looks like!
Note that the URLs provided in this guide point to the Production environment, so make sure you're using the correct one (sandbox/production) accordingly.
Sandbox | Production | |
---|---|---|
API | https://api-sandbox.nofrixion.com | https://api.nofrixion.com |
π» Implementation Guide
βΉοΈ Key concepts
π° Payment Request
A Payment Request is an object that represents the merchant's intention to get paid, similar to an invoice. It contains information such as the amount, currency, and available payment methods. Since the Apple Pay button is the UX/UI representation of a Payment Request, you'll need to create one via an API call from your server, which will be used as a parameter when adding the Apple Pay button to your website.
π Apple Pay Button Visibility
It's important to note that the Apple Pay button is specifically designed for Apple devices using the Safari browser. As a result, the button will only be displayed on compatible devices and browsers. Other browsers and devices will not display the Apple Pay button.
π Apple Domain Verification
Domain verification is a crucial step in the process of implementing Apple Pay on your website. It ensures that your domain is authorised to use Apple Pay, preventing unauthorised domains from implementing the payment method.
π Security
Due to the high security standards required for handling transactions, the Nofrixion Apple Pay Button provides a convenient way to handle sensitive payment details without storing them on merchant systems. When processing an Apple Pay payment, our library communicates directly with our payment gateway, so merchant systems don't need to process or store card details.
π Preconditions
π Verify your domain with Apple
To implement Apple Pay on your website, you must first verify your domain with Apple. This is an essential security measure to ensure that only authorised domains can accept Apple Pay transactions.
Please follow these steps:
- Creating the
.well-known
folder
Before requesting the.txt
file, make sure that your server has a folder named .well-known at its root level. This folder is used to store files related to domain verification and other security measures. If it doesn't already exist, create it at the root level of your domain. - Requesting the
.txt
file
To request the.txt
file, send an email to [email protected], including your fully qualified top-level domain or sub-domain where you will accept Apple Pay (e.g., classbooker.com or pay.classbooker.com). - Placing the
.txt
file on Your Server
Once you receive theapple-developer-merchantid-domain-association.txt
file from us, place it in the.well-known
folder on your server. This file should be publicly accessible, and you should be able to access it at the following URL:https://yourdomain.com/.well-known/apple-developer-merchantid-domain-association.txt
- Informing us of the Completed Verification Process
After placing the file in the appropriate folder, let us know by replying to the email. We will verify the presence of the file and confirm your domain's authorisation to use Apple Pay. Once the verification is complete, you can proceed with implementing the Apple Pay button on your website.
π° Payment Request
As mentioned in the Key Concepts, the NoFrixion Pay Element needs to be linked with a payment request. To create a Payment Request, you need to execute an API call from your system so that you can pass the payment request ID to the Pay Element. If you want to know how to do this, please click click here.
Ensure that you have created a Payment Request with the "Apple Pay" method included
To make your life easier, here's a cURL command that you can copy and paste to create a Payment Request.
You'll need to replace {YOUR_MERCHANT_TOKEN}
with your actual merchant token. Here is how to get your merchant token.
curl -s --location --request POST 'https://api.nofrixion.com/api/v1/paymentrequests' \
--header 'Authorization: Bearer {YOUR_MERCHANT_TOKEN}' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'amount=10.99' \
--data-urlencode 'Currency=EUR' \
--data-urlencode 'PaymentMethodTypes=applePay'
curl -s --location --request POST 'https://api-sandbox.nofrixion.com/api/v1/paymentrequests' \
--header 'Authorization: Bearer {YOUR_MERCHANT_TOKEN}' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'amount=10.99' \
--data-urlencode 'Currency=EUR' \
--data-urlencode 'PaymentMethodTypes=applePay'
π οΈ Step-by-step
-
The entire Apple Pay logic, including security and rendering, is inside the NoFrixion Javascript library. You can find this library in NoFrixion's CDN at https://cdn.nofrixion.com/nofrixion-nextgen.js. Add this library to your
<head>
tag.<head> <meta charset="UTF-8" /> <!-- ππ» Add NoFrixion JavaScript library --> <script src="https://cdn.nofrixion.com/nofrixion-nextgen.js"></script> </head>
-
Add the Apple Pay button to your website by using the
<nofrixion-apple-pay-button />
tagReplace{YOUR_PAYMENT_REQUEST_ID}
with the actual value you got from the Payment Request creation call (please read the Preconditions section) in the code below<body> <!-- ππ» Use the WebComponent where the Apple Pay button will be injected --> <nofrixion-apple-pay-button paymentrequestid="{YOUR_PAYMENT_REQUEST_ID}" apiUrl="https://api.nofrixion.com/api/v1"></nofrixion-apple-pay-button> </body>
<body> <!-- ππ» Use the WebComponent where the Apple Pay button will be injected --> <nofrixion-apple-pay-button paymentrequestid="{YOUR_PAYMENT_REQUEST_ID}" apiUrl="https://api-sandbox.nofrixion.com/api/v1"></nofrixion-apple-pay-button> </body>
Putting all the pieces together, the entire code should look like:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<!-- ππ» Add NoFrixion JavaScript library -->
<script src="https://cdn.nofrixion.com/nofrixion-nextgen.js"></script>
</head>
<body>
<!-- ππ» Use the WebComponent where the Apple Pay button will be injected -->
<nofrixion-apple-pay-button paymentRequestID="{YOUR_PAYMENT_REQUEST_ID}" apiUrl="https://api.nofrixion.com/api/v1"></nofrixion-apple-pay-button>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<!-- ππ» Add NoFrixion JavaScript library -->
<script src="https://cdn.nofrixion.com/nofrixion-nextgen.js"></script>
</head>
<body>
<!-- ππ» Use the WebComponent where the Apple Pay button will be injected -->
<nofrixion-apple-pay-button paymentRequestID="{YOUR_PAYMENT_REQUEST_ID}" apiUrl="https://api-sandbox.nofrixion.com/api/v1"></nofrixion-apple-pay-button>
</body>
</html>
π§ I still have questions
With this implementation, your website is now ready to accept Apple Pay payments from your customers. If you have any questions or issues, please do not hesitate to reach out to our support team for assistance.
Updated 5 months ago